Simple Hibernate Table Creation from Scratch
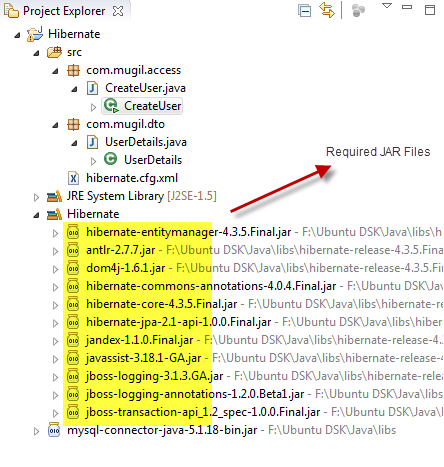
Step1: Create a Bean for which Table should be created in Database
Step2: Create a Class which uses the bean.
Step 1
package com.mugil.dto; import javax.persistence.Entity; import javax.persistence.Id; @Entity public class UserDetails { @Id private int userId; private String userName; public int getUserId() { return userId; } public void setUserId(int userId) { this.userId = userId; } public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } }
Step 2
package com.mugil.access; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; import org.hibernate.cfg.Configuration; import org.hibernate.service.ServiceRegistry; import com.mugil.dto.UserDetails; public class CreateUser { private static ServiceRegistry serviceRegistry; private static SessionFactory sessionFactory; public static void main(String[] args) { UserDetails objUserDetail = new UserDetails(); objUserDetail.setUserId(101); objUserDetail.setUserName("Mugil"); SessionFactory sessionFact = createSessionFactory(); Session session = sessionFact.openSession(); session.beginTransaction(); session.save(objUserDetail); session.getTransaction().commit(); } public static SessionFactory createSessionFactory() { Configuration configuration = new Configuration(); configuration.configure(); serviceRegistry = new StandardServiceRegistryBuilder().applySettings( configuration.getProperties()).build(); sessionFactory = configuration.buildSessionFactory(serviceRegistry); return sessionFactory; } }
In Some cases the hibernate.cfg.xml might become unrecognized.In such case the code should be changed to force the config to be picked from the file location.
public static SessionFactory createSessionFactory() { Configuration configuration = new Configuration().configure(); configuration.configure("hibernate.cfg.xml"); configuration.addAnnotatedClass(com.mugil.tutor.UserDetails.class); serviceRegistry = new StandardServiceRegistryBuilder().applySettings(configuration.getProperties()).build(); sessionFactory = configuration.buildSessionFactory(serviceRegistry); return sessionFactory; }
Hibernate uses SessionFactory pattern internally as below
SessionFactory sessionFact = createSessionFactory(); Session session = sessionFact.openSession(); session.beginTransaction(); session.save(objUserDetail); session.getTransaction().commit();
1.Create Object for SessionFactory
2.Open Session to begin Transaction
3.Begin Transaction using beginTransaction() Method
4.Save the Object by Passing Object of the bean
5.Complete the Transaction using commit
Annotations
@Entity – Means entity as a whole>table would be created by the Name of the Entity
@Id – Tells the Primary Key
Having a Different table name from Class Name
Annotations
@Entity(name="User_Details") public class Users { . . }
Table with User_Details would be created instead of Users
Having a Different Column name from Object Name
Annotations
@Entity(name="User_Details") public class Users { @Id @Column(name="USER_ID") private String UserId; . . }
Columns with User_Id would be created instead of UserId
Appending String to Getters
public void setName(String name) { Name = name + " Append Test "; }
Appending String to Getters
@Entity @Table (name="User_Details") public class Users { }
The Entity Name Still remains the same but the table Name is different.
@Basic Annotation – Tells Hibernate to persist which it does by default
public class Users { @Basic private String UserName; . . }
@Basic has 2 Parameters – Fetch, optional. The only time you use @Basic is while applying the above options.
@Transient Annotation – Tells Hibernate to not store data in database
public class Users { @Transient private String UserName; . . }
@Temporal Annotation – Tells Hibernate to specify Date or Time
public class Users { @Temporal (TemporalType.Date) private String joinedDate; . . }
Without @Temporal the joinedDate is store along with TimeStamp in DB. Now using TemporalType(which is ENUM) you can select the type of data which can be stored in Database.
@Lob – Tells Hibernate to specify Date or Time
public class Users { @Lob private String Address; . . }
Tells the database field should be created as CLOB instead of VARCHAR(255).
Pingback: jesse