- @BeforeAll, @AfterAll – Runs Before and After Class and should be static
- @DisplayName – Displays description about the Test Method
- @Disabled – @Ignore in Junit 4. Disables the Test Method
- @Nested – Helps in grouping of similar test methods together
- @ParameterizedTest – Supplying more than one input for same method in row using array
- @ValueSource – Provides multiple paramters to same test method for ParameterizedTest
- @CsvSource – Provides multiple paramters to same test method for ParameterizedTest in Key Value Pair. Key is Input and Value is expected Output
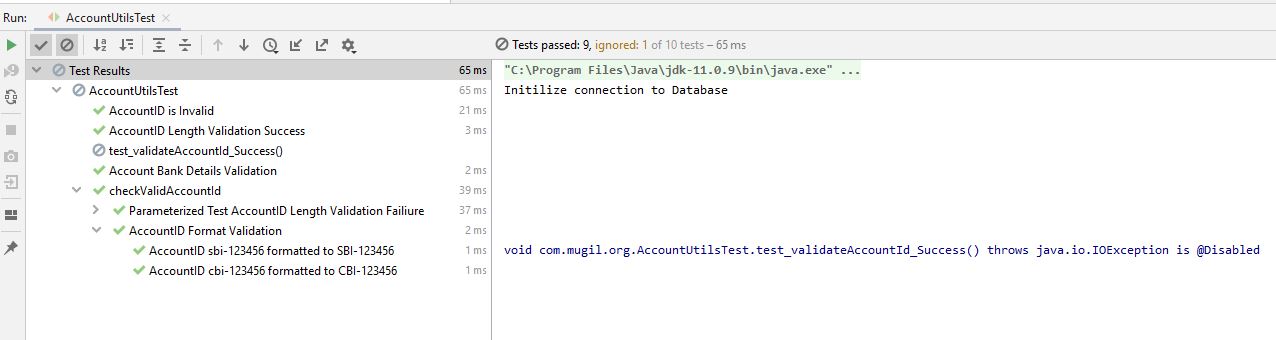
AccountUtils.java
import java.io.IOException;
public class AccountUtils {
public boolean validateAccountId(String acctNo) throws IOException {
if (getAccountIDLength(acctNo)) {
return true;
} else {
throw new IOException("Account ID is Invalid");
}
}
public boolean getAccountIDLength(String acctNo)
{
if(acctNo.length() < 5) return false;
if(acctNo.length() > 10) return false;
if(!acctNo.contains("-")) return false;
return true;
}
public String getFormattedAccID(String accountNo){
return accountNo.toUpperCase();
}
public String[] getBankDetails(String accountNo){
String[] arrAccountDetails = accountNo.split("-");
return arrAccountDetails;
}
}
AccountUtilsTest.java
import org.junit.jupiter.api.*;
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.CsvSource;
import org.junit.jupiter.params.provider.ValueSource;
import java.io.IOException;
import static org.junit.jupiter.api.Assertions.fail;
public class AccountUtilsTest {
AccountUtils systemUnderTest = new AccountUtils();
@BeforeAll
static void init(){
System.out.println("Initilize connection to Database");
}
@AfterAll
static void destroy(){
System.out.println("Deallocate connection to Database");
}
@Test
@DisplayName("AccountID Length Validation Success")
void test_getAccountIDLength_Success(){
boolean expectedOutput = true;
boolean actualOutput = systemUnderTest.getAccountIDLength("SBI-104526");
Assertions.assertEquals(expectedOutput, actualOutput);
Assertions.assertTrue(actualOutput);
}
@Test
@Disabled
void test_validateAccountId_Success() throws IOException {
Assertions.assertTrue(systemUnderTest.validateAccountId("SBI-104526"));
}
@Test
@DisplayName("AccountID is Invalid ")
void test_validateAccountId_Exception(){
Assertions.assertThrows(IOException.class, ()->{
systemUnderTest.validateAccountId("");
});
}
@Test
@DisplayName("Account Bank Details Validation")
void test_AccountIDForBankName_Success(){
String[] expectedOutput = new String[]{"SBI", "104526"};
String[] actualOutput = systemUnderTest.getBankDetails("SBI-104526");
Assertions.assertArrayEquals(expectedOutput, actualOutput);
}
@Nested
class checkValidAccountId
{
@ParameterizedTest
@ValueSource(strings={"SBI", "SBI-123456789", " ", ""})
@DisplayName("Parameterized Test AccountID Length Validation Failiure")
void test_getAccountIDLength_Failiure(String accountId){
Assertions.assertFalse(systemUnderTest.getAccountIDLength(accountId));
}
@ParameterizedTest(name="AccountID {0} formatted to {1}")
@CsvSource(value={"sbi-123456, SBI-123456", "cbi-123456, CBI-123456"})
@DisplayName("AccountID Format Validation")
void getFormattedAccID_Success(String inputString, String expectedOutput){
Assertions.assertEquals(expectedOutput, systemUnderTest.getFormattedAccID(inputString));
}
}
}