In a Application the persistence unit used to store the data may vary.Accessing data varies depending on the source of the data. Access to persistent data varies greatly depending on the type of storage (database, flat files, xml files, and so on) and it even differs from its implementation (for example different SQL-dialects).
The advantage of the DAO layer is that if you need to change the underlying persistence mechanism you only have to change the DAO layer, and not all the places in the domain logic where the DAO layer is used from. The DAO layer usually consists of a smaller set of classes, than the number of domain logic classes that uses it.
Abstract and encapsulate all access to the data and provide an interface. This is called the Data Access Object pattern. In a nutshell, the DAO “knows” which data source (that could be a database, a flat file or even a WebService) to connect to and is specific for this data source (e.g. a OracleDAO might use oracle-specific data types, a WebServiceDAO might parse the incoming and outgoing message etc.)
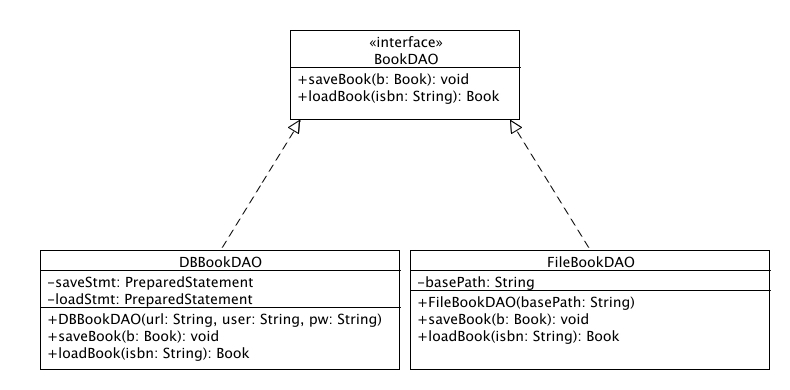
BookDAO.java
public interface BookDAO {
public void saveBook(Book b);
public Book loadBook(String isbn);
}
DBBookDAO.java
public class DBBookDAO implements BookDAO {
private PreparedStatement saveStmt;
private PreparedStatement loadStmt;
public DBBookDAO(String url, String user, String pw) {
Connection con = DriverManager.getConnection(url, user, pw);
saveStmt = con.prepareStatement("INSERT INTO books(isbn, title, author) "
+"VALUES (?, ?, ?)");
loadStmt = con.prepareStatement("SELECT isbn, title, author FROM books "
+"WHERE isbn = ?");
}
public Book loadBook(String isbn) {
Book b = new Book();
loadStmt.setString(1, isbn);
ResultSet result = loadStmt.executeQuery();
if (!result.next()) return null;
b.setIsbn(result.getString("isbn"));
b.setTitle(result.getString("title"));
b.setAuthor(result.getString("author"));
return b;
}
public void saveBook(Book b) {
saveStmt.setString(1, b.getIsbn());
saveStmt.setString(2, b.getTitle());
saveStmt.setString(3, b.getAuthor());
saveStmt.executeUpdate();
}
}
FileBookDAO.java
public class FileBookDAO implements BookDAO {
private String basePath;
public FileBookDAO(String basePath) {
this.basePath = basePath;
}
public Book loadBook(String isbn) {
FileReader fr = new FileReader(basePath + isbn);
BufferedReader br = new BufferedReader(fr);
Book b = new Book();
String rIsbn = br.readLine();
String rTitle = br.readLine();
String rAuthor = br.readLine();
if (rIsbn.startsWith("ISBN: ")) {
b.setIsbn(rIsbn.substring("ISBN: ".length()));
} else {
return null;
}
if (rTitle.startsWith("TITLE: ")) {
b.setTitle(rTitle.substring("TITLE: ".length()));
} else {
return null;
}
if (rAuthor.startsWith("AUTHOR: ")) {
b.setAuthor(rAuthor.substring("AUTHOR: ".length()));
} else {
return null;
}
return b;
}
public void saveBook(Book b) {
FileWriter fw = new FileWriter(basePath + b.getIsbn() + ".book");
fw.write("ISBN: " + b.getIsbn());
fw.write("TITLE: " + b.getTitle());
fw.write("AUTHOR: " + b.getAuthor());
fw.close();
}
}